I very luckily got landed with a:
I wired the Tripwire sensor to the Phidget board as described in the wiring diagram and as shown in the video:
I connected the USB cable from the Phidgets Board to the USB port on a Raspberry Pi 3 and proceeded to compile the Phidget libraries on the Pi (info):
sudo apt-get install libusb-1.0-0-dev wget http://www.phidgets.com/downloads/libraries/libphidget.tar.gz tar xzf libphidget.tar.gz cd libphidget-2.1.8.20151217 ./configure make sudo make install
I then tested some C code examples:
wget http://www.phidgets.com/downloads/examples/phidget21-c-examples.tar.gz tar xzf phidget21-c-examples.tar.gz cd phidget21-c-examples-2.1.8.20151217 gcc HelloWorld.c -o HelloWorld -lphidget21 sudo ./HelloWorld
That successfully showed the Phidgets Board serial number so I moved on to the Python libraries:
wget http://www.phidgets.com/downloads/libraries/PhidgetsPython.zip unzip PhidgetsPython.zip cd PhidgetsPython sudo python setup.py install
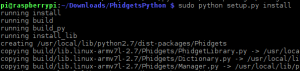
With the python libraries installed I downloaded and tested a sample Python script:
wget http://www.phidgets.com/downloads/examples/Python.zip unzip Python.zip cd Python sudo python HelloWorld.py
sudo python InterfaceKit-simple.py
This showed details of the Phidget Board including sensors connected to the Input/Output pins. When I activated and tripped the tripwire I could see the True/False outputs as follows:
Next I amended the InterfaceKit-simple.py script to print out a message when the sensor was tripped and to play a sound file. This could obviously be improved but is a starting point for what we are aiming to do.
#!/usr/bin/env python """Copyright 2010 Phidgets Inc. This work is licensed under the Creative Commons Attribution 2.5 Canada License. To view a copy of this license, visit http://creativecommons.org/licenses/by/2.5/ca/ """ __author__ = 'Adam Stelmack' __version__ = '2.1.8' __date__ = 'May 17 2010' #Basic imports from ctypes import * import sys import random import pygame #Phidget specific imports from Phidgets.PhidgetException import PhidgetErrorCodes, PhidgetException from Phidgets.Events.Events import AttachEventArgs, DetachEventArgs, ErrorEventArgs, InputChangeEventArgs, OutputChangeEventArgs, SensorChangeEventArgs from Phidgets.Devices.InterfaceKit import InterfaceKit from Phidgets.Phidget import PhidgetLogLevel #Create an interfacekit object try: interfaceKit = InterfaceKit() except RuntimeError as e: print("Runtime Exception: %s" % e.details) print("Exiting....") exit(1) #Information Display Function def displayDeviceInfo(): print("|------------|----------------------------------|--------------|------------|") print("|- Attached -|- Type -|- Serial No. -|- Version -|") print("|------------|----------------------------------|--------------|------------|") print("|- %8s -|- %30s -|- %10d -|- %8d -|" % (interfaceKit.isAttached(), interfaceKit.getDeviceName(), interfaceKit.getSerialNum(), interfaceKit.getDeviceVersion())) print("|------------|----------------------------------|--------------|------------|") print("Number of Digital Inputs: %i" % (interfaceKit.getInputCount())) print("Number of Digital Outputs: %i" % (interfaceKit.getOutputCount())) print("Number of Sensor Inputs: %i" % (interfaceKit.getSensorCount())) #Event Handler Callback Functions def interfaceKitAttached(e): attached = e.device print("InterfaceKit %i Attached!" % (attached.getSerialNum())) def interfaceKitDetached(e): detached = e.device print("InterfaceKit %i Detached!" % (detached.getSerialNum())) def interfaceKitError(e): try: source = e.device print("InterfaceKit %i: Phidget Error %i: %s" % (source.getSerialNum(), e.eCode, e.description)) except PhidgetException as e: print("Phidget Exception %i: %s" % (e.code, e.details)) def interfaceKitInputChanged(e): source = e.device print("InterfaceKit %i: Input %i: %s" % (source.getSerialNum(), e.index, e.state)) if e.index == 3 and e.state == True: print(" ----- TRIPPED ----- ") # Prepare the sound file pygame.mixer.init() pygame.mixer.music.load("fart.wav") pygame.mixer.music.play() def interfaceKitSensorChanged(e): source = e.device print("InterfaceKit %i: Sensor %i: %i" % (source.getSerialNum(), e.index, e.value)) def interfaceKitOutputChanged(e): source = e.device print("InterfaceKit %i: Output %i: %s" % (source.getSerialNum(), e.index, e.state)) #Main Program Code try: #logging example, uncomment to generate a log file #interfaceKit.enableLogging(PhidgetLogLevel.PHIDGET_LOG_VERBOSE, "phidgetlog.log") interfaceKit.setOnAttachHandler(interfaceKitAttached) # interfaceKit.setOnDetachHandler(interfaceKitDetached) interfaceKit.setOnErrorhandler(interfaceKitError) interfaceKit.setOnInputChangeHandler(interfaceKitInputChanged) interfaceKit.setOnOutputChangeHandler(interfaceKitOutputChanged) interfaceKit.setOnSensorChangeHandler(interfaceKitSensorChanged) except PhidgetException as e: print("Phidget Exception %i: %s" % (e.code, e.details)) print("Exiting....") exit(1) print("Opening phidget object....") try: interfaceKit.openPhidget() except PhidgetException as e: print("Phidget Exception %i: %s" % (e.code, e.details)) print("Exiting....") exit(1) print("Waiting for attach....") try: interfaceKit.waitForAttach(10000) except PhidgetException as e: print("Phidget Exception %i: %s" % (e.code, e.details)) try: interfaceKit.closePhidget() except PhidgetException as e: print("Phidget Exception %i: %s" % (e.code, e.details)) print("Exiting....") exit(1) print("Exiting....") exit(1) else: # displayDeviceInfo() print("Would normally display device ino but not today ") print("Setting the data rate for tripwire sensor 3 to 4ms....") try: interfaceKit.setDataRate(3, 4) except PhidgetException as e: print("Phidget Exception %i: %s" % (e.code, e.details)) #print("Setting the data rate for each sensor index to 4ms....") #for i in range(interfaceKit.getSensorCount()): # try: # # interfaceKit.setDataRate(i, 4) # except PhidgetException as e: # print("Phidget Exception %i: %s" % (e.code, e.details)) print("Press Enter to quit....") chr = sys.stdin.read(1) print("Closing...") try: interfaceKit.closePhidget() except PhidgetException as e: print("Phidget Exception %i: %s" % (e.code, e.details)) print("Exiting....") exit(1) print("Done.") exit(0)